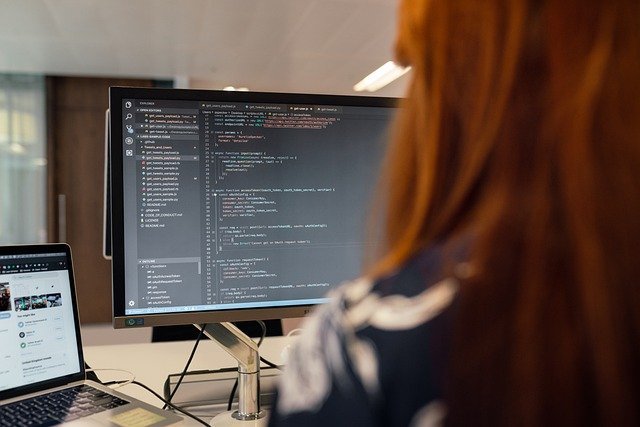
React is one of the most popular web frameworks in the world. Every React project requires expert-level React developers to know React-related concepts. React experts must be familiar with as many React concepts as possible. In this article, we will list the top three coding challenges for React experts. These include creating a Higher-Order Component to reuse component logic. Reusing code is an important skill that React experts should have. The interviewer wants to check how you implement and use Redux in a React application.
Michael Pautov
Software Engineer
Today, React is one of the most popular web frameworks. More and more companies are either using React or switching to React. Every React project requires expert-level React developers.
The React Library is huge and has many concepts. A React expert needs to be familiar with as many React and React-related concepts as possible. Not only should the developer be familiar with all these concepts, he/she should also know how to use them in real-time projects.
Expert-level React interviews are not easy. The coding challenges given to the developer generally consist of advanced-level concepts which could only be solved if the developer has the relevant working experience. In this article, we will list the top 3 coding challenges for React experts.
Create a Higher-Order Component to reuse component logic
Higher-Order Component is an advanced technique used by developers for reusing a component’s logic. Reusing code is an important skill that React experts should have. The major reason to have reusability is code optimization.
In this coding challenge, you might be asked to create three different components that have similar component logic. So you have to create a Higher-Order Component that will have the component logic and it will be reused by the other three components.
For this challenge, you have three components, each containing a button that increments the value in the state by a specific number. Suppose, three components are:
- “ComponentA” where the button increments the value by two.
- “ComponentB” where the button increments the value by twenty.
- “ComponentC” where the button increments the value by two hundred.
First, create a HOC with the logic.
import { useState } from "react";
const HigherOrderComponent = (Component, incrementValue) => {
const HOCFun = () => {
const [value, setValue] = useState(0);
const incrementHandler = () => {
setValue(value + incrementValue);
};
return <Component value={value} incrementHandler={incrementHandler} />;
};
return HOCFun;
};
export default HigherOrderComponent;
“HigherOrderComponent” takes two arguments – a component and the number by which the state will be incremented. Then, a function is created that has the component logic. The logic contains a state variable whose value is incremented by a handler using the incoming number.
This function is returning the incoming component with props – value and incrementHandler. Remember, this is the new component made using the HOC. In the end, this function is returned because it will be used in the existing components.
Now, let’s use HOC in “ComponentA”, “ComponentB”, and “ComponentC”.
ComponentA:
import HigherOrderComponent from "./HigherOrderComponent";
const ComponentA = ({ value, incrementHandler }) => {
return (
<div>
<button onClick={incrementHandler}>Increment by 2</button>
<h2>{value}</h2>
</div>
);
};
export default HigherOrderComponent(ComponentA, 2);
ComponentB:
import HigherOrderComponent from "./HigherOrderComponent";
const ComponentB = ({ value, incrementHandler }) => {
return (
<div>
<button onClick={incrementHandler}>Increment by 29</button>
<h2>{value}</h2>
</div>
);
};
export default HigherOrderComponent(ComponentB, 20);
ComponentC:
import HigherOrderComponent from "./HigherOrderComponent";
const ComponentC = ({ value, incrementHandler }) => {
return (
<div>
<button onClick={incrementHandler}>Increment by 200</button>
<h2>{value}</h2>
</div>
);
};
export default HigherOrderComponent(ComponentC, 200);
None of these components contain any logic but still, everything’s working.
This happens because a Higher-Order Component is being used for code reusability.
Now, remember, the motive for this coding challenge is to check how you create the Higher-Order Component and reuse the logic.
Implementing and using Redux
When the application grows, managing the global state becomes tough. Redux is the most popular third-party library used for state management with React. An expert React developer should understand what Redux is and how it works. So the interview can ask you to implement Redux in a basic React application.
In this coding challenge, the interviewer wants to check how you implement and use Redux. So, you might be provided with a basic React application with two components – one that will contain the buttons to increment and decrement the global state and another to display the value.
First, create the reducer.
export const reducer = (state = { value: 0 }, action) => {
switch (action.type) {
case "INCREMENT_VALUE":
return {
...state,
value: action.payload + 1,
};
case "DECREMENT_VALUE":
return {
...state,
value: action.payload - 1,
};
default:
return { ...state };
}
};
Along with type, the reducer will also receive a payload from the action.
Then, create action creators. You can also create normal action but creating action creators indicates that you have worked with complex Redux.
export const incrementValueAction = (value) => {
return {
type: "INCREMENT_VALUE",
payload: value,
};
};
export const decrementValueAction = (value) => {
return {
type: "DECREMENT_VALUE",
payload: value,
};
};
Next, create the store.
import { createStore } from "redux";
import { reducer } from "./Reducers/reducers";
const initialState = {
value: 0,
};
const store = createStore(reducer, initialState);
export default store;
In the end, wrap the application with Provider for the store.
import { Provider } from "react-redux";
import store from "./store";
import Component1 from "./Components/Component1";
import Component2 from "./Components/Component2";
function App() {
return (
<Provider store={store}>
<div className="App">
<Component1 />
<hr />
<Component2 />
</div>
</Provider>
);
}
export default App;
The first half is ready. Redux is implemented but the job is not complete because using it in React components is still pending. For that, we will use the react-redux hooks. Remember, do not use the older connect() function.
First, install “react-redux”, then use the useDispatch and useSelector react-redux hooks in the components.
Component1:
import { useDispatch, useSelector } from "react-redux";
import {
decrementValueAction,
incrementValueAction,
} from "../ActionCreators/actionCreators";
const Component1 = () => {
const dispatch = useDispatch();
const value = useSelector((state) => state.value);
console.log(value);
const incrementHandler = () => {
dispatch(incrementValueAction(value));
};
const decrementHandler = () => {
dispatch(decrementValueAction(value));
};
return (
<div>
<button onClick={incrementHandler}>Increment</button>
<button onClick={decrementHandler}>Decrement</button>
</div>
);
};
export default Component1;
Component2:
import { useSelector } from "react-redux";
const Component2 = () => {
const value = useSelector((state) => state.value);
return (
<div>
<h2>{value}</h2>
<hr />
</div>
);
};
export default Component2;
With react-redux hooks in use, buttons will work.
Now, the main motive is to check your redux knowledge. The interview may make this challenge tougher by asking you to use redux-thunk in it. Moreover, use the react-redux hooks to give a better impression and avoid older techniques.
Share data among components without using props
In this coding challenge, the interview might give you a React application with multiple nested components like the following.
A
|
B
|
————–
| |
C D
Component “B” is the child component of “A” while component “C” and “D” are child components of “B”.
Suppose there is an object in component “A” and it is required in “C” and “D”. There are two ways to share this object in these nested components without using props. The first is by using Redux. But never use Redux in such cases where the interviewer wants to avoid props because Redux is meant for complex projects. Actually, the interviewer is expecting “Context” for this coding challenge.
For this challenge, first, create a context.
import React from "react";
const DemoContext = React.createContext();
export default DemoContext;
Then, using this context, wrap the component tree in a Provider.
import DemoContext from "../DemoContext";
import B from "./B";
const A = () => {
const obj = {
a: 1,
b: 2,
c: 3,
};
return (
<DemoContext.Provider value={{ obj }}>
<div>
<B />
</div>
</DemoContext.Provider>
);
};
export default A;
Now, we can access the “obj” in components “C” and “D”. There are two ways for consuming the context – by using the Consumer and useContext hook. Prefer using the useContext hook because it is the modern and better way.
C:
import React, { useContext } from "react";
import DemoContext from "../DemoContext";
const C = () => {
const { obj } = useContext(DemoContext);
const { a, b, c } = obj;
return (
<div>
<h2>Component C</h2>
<h3>{a}</h3>
<h3>{b}</h3>
<h3>{c}</h3>
</div>
);
};
export default C;
D:
import React, { useContext } from "react";
import DemoContext from "../DemoContext";
const D = () => {
const { obj } = useContext(DemoContext);
const { a, b, c } = obj;
return (
<div>
<h2>Component D</h2>
<h3>{a}</h3>
<h3>{b}</h3>
<h3>{c}</h3>
</div>
);
};
export default D;
Let’s check the output.
It’s working without using props!
Wrapping it up
Coding challenges for expert React developers can be tough. The interviewer wants to check your knowledge of React as well as your working experience. So the challenges will have advanced-level concepts such as HOC, Redux, and Context.
Tags
Source: https://hackernoon.com/top-3-coding-challenges-for-expert-level-react-developers